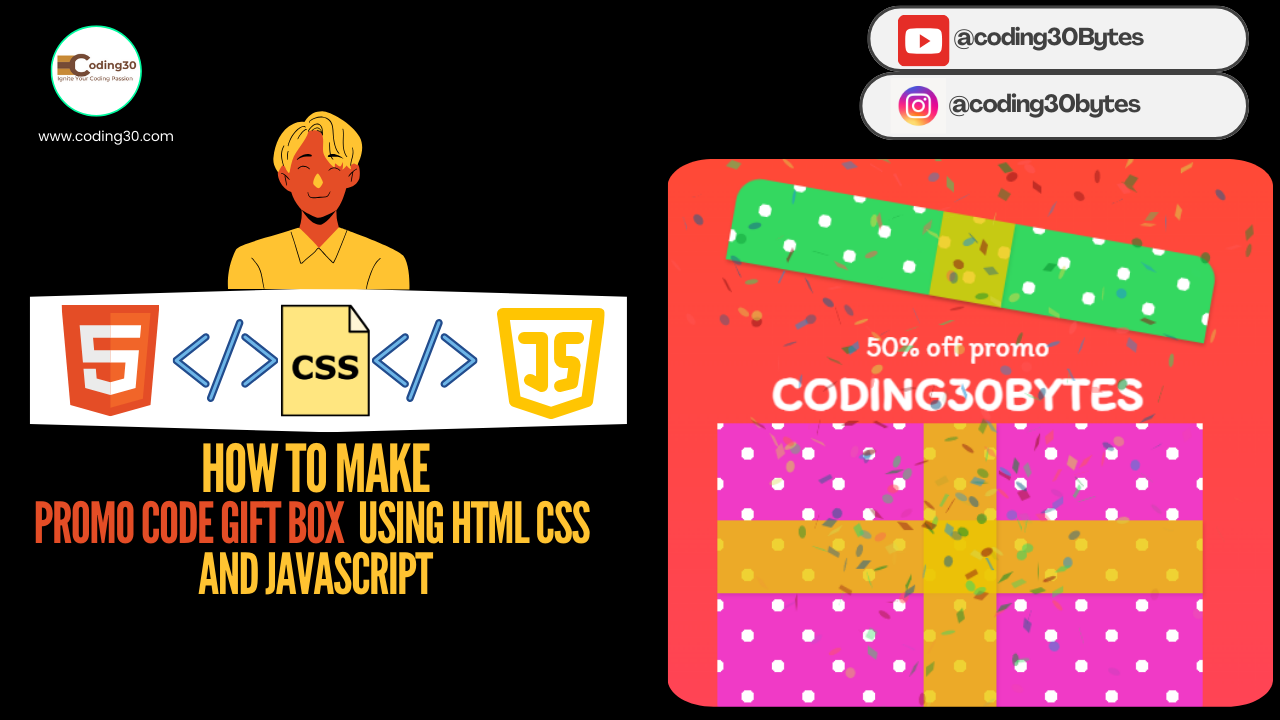
Introduction:
Hello friends, today I will create promo code gift box. To create a promo code gift box, use HTML to structure the box and CSS for styling. The box should have a distinct appearance with a background color, padding, and borders to resemble a gift box. Add a ribbon using pseudo-elements and style the text to display the promo code prominently. Include hover effects to make the box interactive, giving a pleasant user experience.
This is the index.html file code.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>coding30 | Promo Code</title>
</head>
<body>
<div class="present" id="present">
<div class="lid">
<span></span>
</div>
<div class="promo">
<p>50% off promo</p>
<h2>CODING30BYTES</h2>
</div>
<div class="box">
<span></span>
<span></span>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/canvas-confetti@1.4.0/dist/confetti.browser.min.js"></script>
<script src="script.js"></script>
</body>
</html>
This is the style.css file code.
@import url("https://fonts.googleapis.com/css2?family=Itim&display=swap");
* {
box-sizing: border-box;
}
body {
background: #232526;
background: linear-gradient(to top,
#FF416C, #FF4B2B);
font-family: "Itim", cursive;
min-height: 100vh;
margin: 0;
display: flex;
justify-content: center;
align-items: center;
}
.present {
width: 410px;
max-width: 90vw;
margin: 0 auto;
cursor: pointer;
animation: bounce 3s ease-in-out;
}
.box {
width: 400px;
max-width: 90vw;
height: 250px;
border-bottom-left-radius: 20px;
border-bottom-right-radius: 20px;
}
.box,
.lid {
background: radial-gradient(white 15%,
transparent 15.1%),
radial-gradient(white 15%, transparent 15.1%),
rgb(240, 58, 198);
background-position: 0 0, 25px 25px;
background-size: 50px 50px;
position: relative;
margin: 0 auto;
}
.lid {
width: 400px;
max-width: 90vw;
height: 70px;
box-shadow: 1px 2px 3px rgba(0, 0, 0, 0.2);
z-index: 1;
padding: 0 2px;
background-color: rgb(52, 216, 96);
top: 0;
left: 0;
transition: top ease-out 0.5s, left ease-out 0.5s,
transform ease-out 0.5s;
border-top-left-radius: 20px;
border-top-right-radius: 20px;
}
.box span,
.lid span {
position: absolute;
display: block;
background: rgba(235, 199, 0, 0.8);
box-shadow: 1px 2px 3px rgba(0, 0, 0, 0.1);
}
.box span:first-child {
width: 100%;
height: 60px;
top: 80px;
}
.box span:last-child,
.lid span {
width: 60px;
height: 100%;
left: 170px;
}
.promo {
font-size: 30px;
color: white;
text-align: center;
position: relative;
height: 0;
top: 10px;
transition: all ease-out 0.7s;
}
.promo p {
font-size: 24px;
margin: 0;
}
.promo h2 {
font-size: 40px;
margin: 0;
}
.present:hover .lid {
top: -100px;
transform: rotateZ(10deg);
left: 10px;
}
.present:hover .promo {
top: -80px;
animation: vibrate 0.3s infinite;
}
@keyframes vibrate {
0% { transform: translate(0); }
20% { transform: translate(-2px, 2px); }
40% { transform: translate(-2px, -2px); }
60% { transform: translate(2px, 2px); }
80% { transform: translate(2px, -2px); }
100% { transform: translate(0); }
}
@keyframes bounce {
0%, 20%, 50%, 80%, 100% {
transform: translateY(0);
}
40% {
transform: translateY(-30px);
}
60% {
transform: translateY(-15px);
}
}
This is the script.js file code.
const present = document.getElementById("present");
const options = {
colors: [
"#34D963",
"#068C2C",
"#FF5757",
"#8C1414",
"#D9D74A",
"#1E91D9",
"#1B608C",
],
};
present.addEventListener("mouseenter", () =>{
confetti(options);
})
present.addEventListener("touchstart", () =>{
confetti(options);
})
Video tutorial here...
Preview...
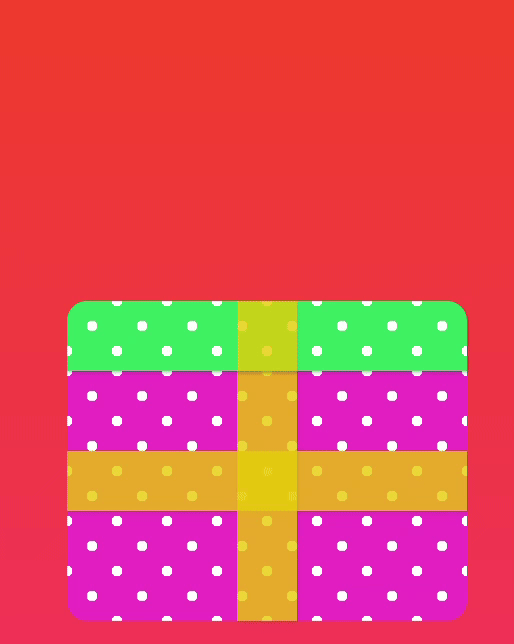