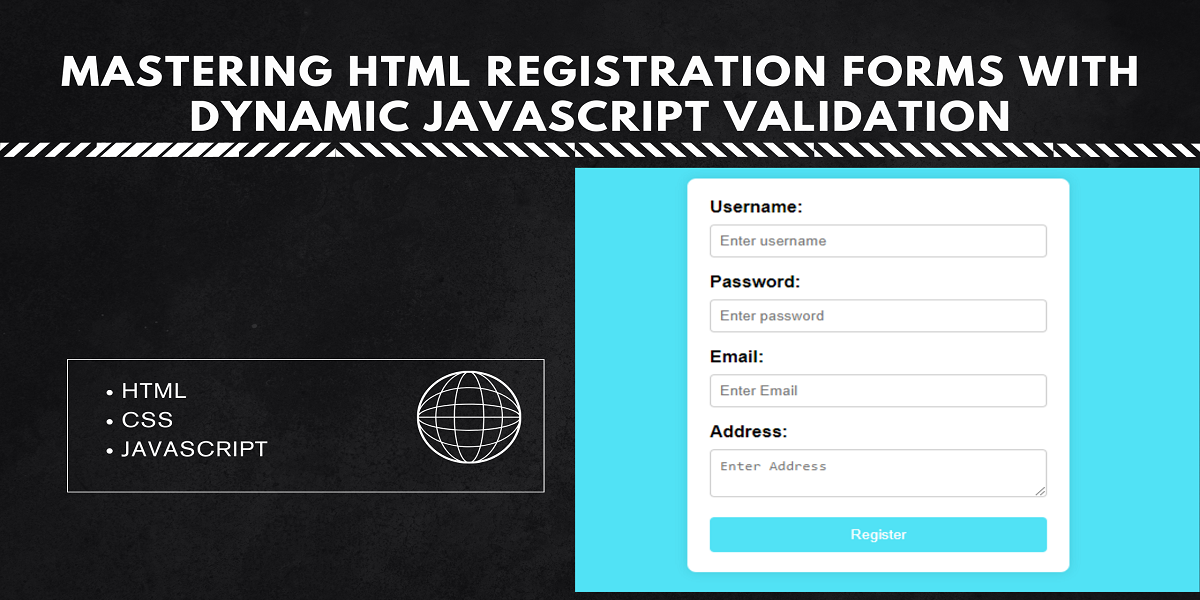
Introduction:
Welcome to a guide that explores the art of creating HTML registration forms with JavaScript validation—a crucial aspect of web development that ensures not only a seamless user experience but also robust security. In this blog post, we'll delve into the process of building registration forms that not only look good but also validate user input in real-time, providing instant feedback and enhancing the overall user experience.
1. Understanding the Significance of Form Validation:
- HTML form validation assists users in avoiding common input mistakes, such as entering letters in a numeric field or forgetting to fill in required information. This proactive approach minimizes the occurrence of preventable errors.
- Validation messages provide instant feedback to users, guiding them through the correct input format. This real-time interaction significantly enhances the overall user experience, reducing frustration and errors in the form submission process.
- Validating user inputs on the client side before submitting data to the server helps optimize server resources. By filtering out invalid data early in the process, unnecessary server requests and processing are minimized.
Code Block Example
This is the simple html registration form
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Registration Form</title>
<style>
body {
font-family: Arial, sans-serif;
background-color: #51e2f5;
margin: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
#custom_container {
background-color: #fff;
padding: 20px;
border-radius: 8px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
width: 300px;
}
label {
display: block;
margin-bottom: 8px;
font-weight: bold;
}
input {
width: 100%;
padding: 8px;
margin-bottom: 16px;
box-sizing: border-box;
border: 1px solid #ccc;
border-radius: 4px;
}
textarea {
width: 100%;
padding: 8px;
margin-bottom: 16px;
box-sizing: border-box;
border: 1px solid #ccc;
border-radius: 4px;
}
button {
background-color: #51e2f5;
color: #fff;
padding: 10px;
border: none;
border-radius: 4px;
cursor: pointer;
width: 100%;
}
button:hover {
background-color: #51e2f5;
}
</style>
</head>
<body>
<div id="custom_container">
<label for="username">Username:</label>
<input type="text" id="username" name="username" placeholder="Enter username" required>
<label for="password">Password:</label>
<input type="password" id="password" name="password" placeholder="Enter password" required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" placeholder="Enter Email" required>
<label for="text_area">Address:</label>
<textarea id="text_area" name="text_area" placeholder="Enter Address" required></textarea>
<button onclick="submit_form()">Register</button>
</div>
<script src="sweetalert.js"></script>
<script>
function submit_form()
{
var username=document.getElementById("username").value;
var password=document.getElementById("password").value;
var email=document.getElementById("email").value;
var text_area=document.getElementById("text_area").value;
if(username=="")
{
swal("Username can't be empty","","warning").then(function() {});
return;
}
if(password=="")
{
swal("password can't be empty","","warning").then(function() {});
return;
}
if(email=="")
{
swal("Email can't be empty","","warning").then(function() {});
return;
}
if(text_area=="")
{
swal("Address can't be empty","","warning").then(function() {});
return;
}
console.log(username+""+password+""+email+""+text_area);
var data='{"username":"'+username+'","password":"'+password+'","email":"'+email+'","text_area":"'+text_area+'"}';
var xhr = new XMLHttpRequest();
xhr.open("POST","/enter your api url",true);
xhr.setRequestHeader("Content-Type", "application/json","Access-Control-Allow-Origin","*");
xhr.onload = function () {
data=JSON.parse(this.responseText);
if(data.status=="200"){
swal("Successfully","Registration!!","success").then(function() {
location.reload();
});
}else{
swal(data.message,"","warning").then(function() {
});
}
}
xhr.send(data);
}
</script>
</body>
</html>
HTML DOM Document getElementById()
if you want get html "input text box value" then you can use getElementById() for access the input box value.
function getUsername{
var username=document.getElementById(username).value;
// print user input name and data will show in console
console.log("your user input:::"+username);
}
XMLHttpRequest Object
- XMLHttpRequest Object can be used request data form server.
- XMLHttpRequest Object use you can hit rest api and get response via webserver.
- You can send data to webser in the background.
function HitRestApiREquest {
var xhr = new XMLHttpRequest();
xhr.open("POST","/enter your api url",true);
xhr.setRequestHeader("Content-Type", "application/json","Access-Control-Allow-Origin","*");
xhr.onload = function () {
data=JSON.parse(this.responseText);
if(data.status=="200"){
swal("Successfully","Registration!!","success").then(function() {
location.reload();
});
}else{
swal(data.message,"","warning").then(function() {
});
}
}
xhr.send(data);
}
Conclusion:
- Html with javascript is the best combination of making registration form. This is very easy to use. if user enter wrong input in text box like username,password e.t.c. then java script validation is very fast work and show alert popup message.