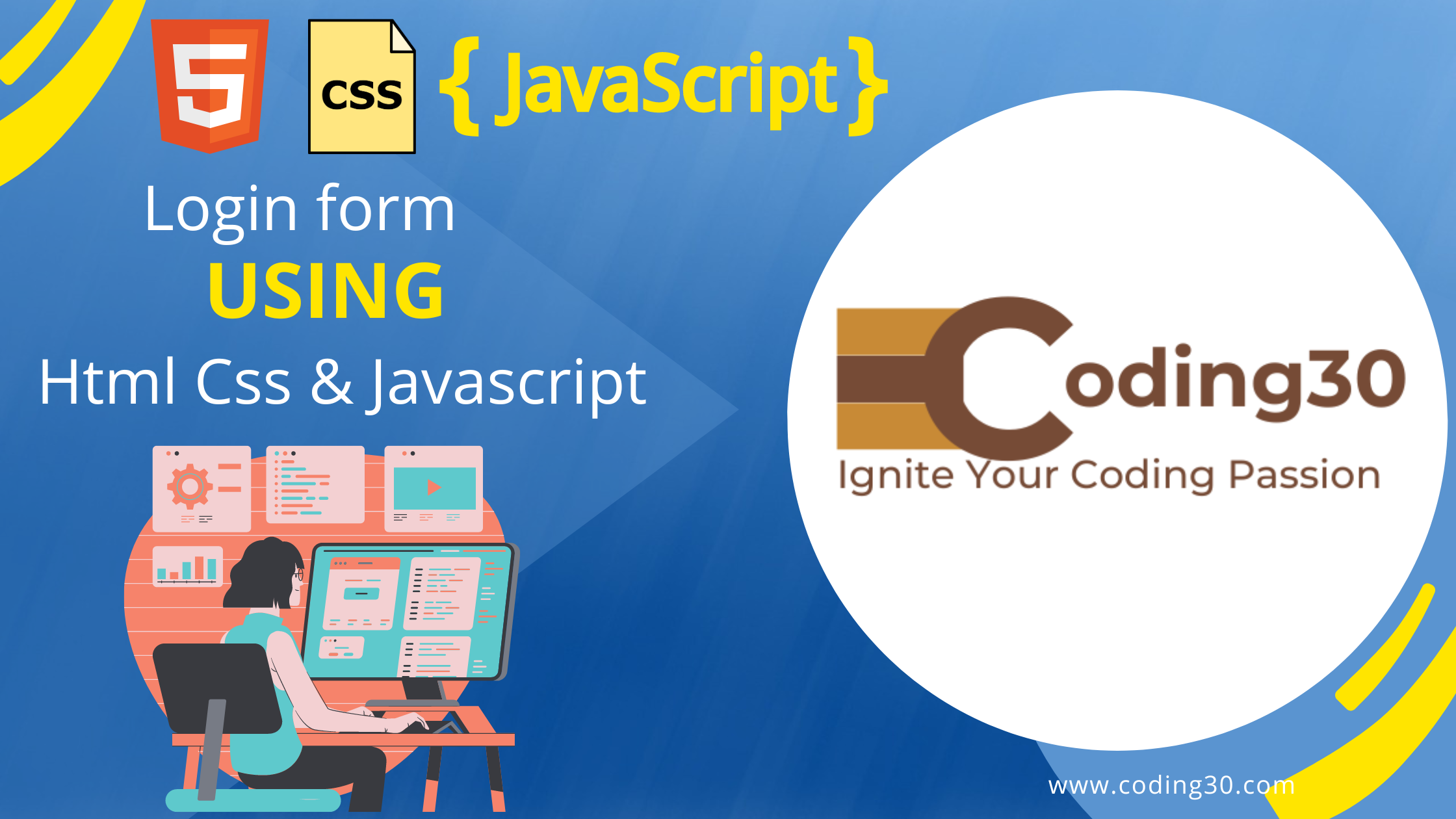
Introduction:
A login form allows users to enter credentials to access a website. HTML structures the form with input fields for the username and password, and a submit button. CSS styles the form for a user-friendly appearance. JavaScript handles form validation and submission.
This is the code...
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>coding30 | User Log in</title>
<!-- Tell the browser to be responsive to screen width -->
<meta content="width=device-width, initial-scale=1, maximum-scale=1, user-scalable=no" name="viewport">
<!-- Font Awesome -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.5.0/css/font-awesome.min.css">
<link href="https://fonts.googleapis.com/css?family=Roboto" rel="stylesheet">
<!-- Bootstrap core CSS -->
<link href="bootstrap5.css" rel="stylesheet">
<link href="style.css" rel="stylesheet">
</head>
<body>
<!-- Navbar coding start -->
<nav class="navbar navbar-expand-lg navbar-light bg-light" style="background-color: #CD5C5C !important;">
<div class="container-fluid">
<a class="navbar-brand text-white" href="#">Logo</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav">
<li class="nav-item">
<a class="nav-link active text-white" aria-current="page" href="#">Home</a>
</li>
<li class="nav-item">
<a class="nav-link text-white" href="#">Features</a>
</li>
<li class="nav-item">
<a class="nav-link text-white" href="#">Pricing</a>
</li>
</ul>
</div>
</div>
</nav>
<!-- Navbar coding end -->
<div class="container">
<div class="modal fade" id="css_loader" tabindex="-1" role="dialog" aria-labelledby="exampleModalLabel" aria-hidden="true" data-bs-keyboard="false" data-bs-backdrop="static">
<div class="modal-dialog modal-sm" role="document">
<div class="modal-content">
<div class="modal-body" style="text-align: center;">
<div class="loader"></div>
<span>Please wait...</span>
</div>
</div>
</div>
</div>
<div class="row">
<div class="col-lg-3 col-md-2"></div>
<div class="col-lg-6 col-md-8 login-box">
<div class="col-lg-12 login-key">
<img src="admin_icon.png"/>
</div>
<div class="col-lg-12 login-title">
USER PANEL
</div>
<div class="col-lg-12 login-form">
<div class="col-lg-12 login-form">
<div class="form-group">
<label class="form-control-label">USERNAME</label>
<input type="text" id="username" placeholder="Enter user name" class="form-control">
</div>
<div class="form-group">
<label class="form-control-label" >PASSWORD</label>
<input type="password" id="password" placeholder="Enter password" class="form-control" i>
</div>
<div class="col-lg-12 loginbttm">
<div class="col-lg-6 login-btm login-text">
<!-- Error Message -->
</div>
<div class="col-lg-6 login-btm login-button">
<button type="button" class="btn btn-warning btn-lg" style="color:#ffffff" onclick="login()">LOGIN</button>
</div>
</div>
</div>
</div>
<div class="col-lg-3 col-md-2"></div>
</div>
</div>
<script src="sweetalert.js"></script>
<script src="jquery_slim.js"></script>
<script src="bootstrap_min.js" ></script>
<script>
$('#css_loader').modal('hide');
// This the submit form function
function login() {
$('#css_loader').modal('show');
var username = document.getElementById("username").value;
var password = document.getElementById("password").value;
console.log(username+":::"+password);
if(username ==""){
swal("User name can't be empty","", "warning").then(function() {
$('#css_loader').modal('hide');
});
return;
}
if(password ==""){
swal("Password can't be empty","", "warning").then(function() {
$('#css_loader').modal('hide');
});
return;
}
swal("Login success", "", "success").then(function() {
$('#css_loader').modal('hide');
});
}
</script>
</body>
</html>
Video tutorial here...
Preview...
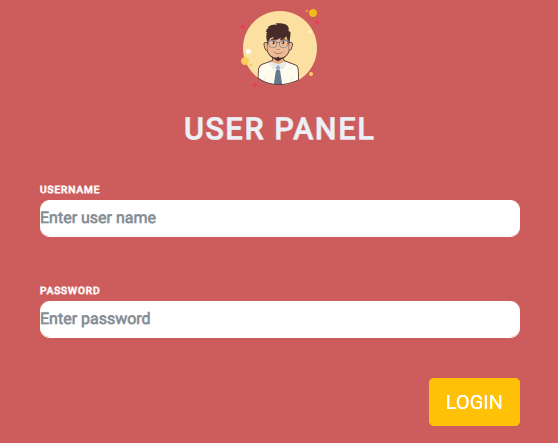
Explanation:
HTML
In the code, we create two input fields using HTML tags one for the username and one for the password.CSS
In the code, CSS styles the form for a user-friendly appearance, styling the form's input fields, submit button, input field borders, and form background color.Javascript
In the code, Javascript using for perform the click action. if user enter the login credentials and click the login button and then user successfully login. if user before filing the login credentials hit the login button then appear the login error popup. so javascript is the very useful for perform the form action like call login function, change button clor, handle the validation and more.