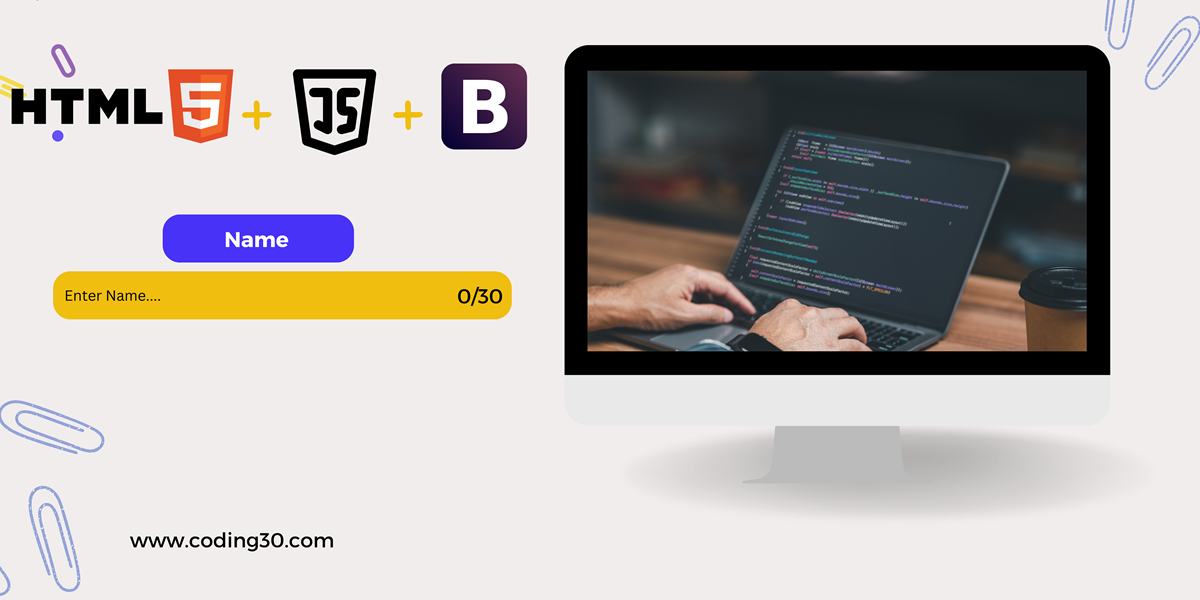
Introduction:
To create an input field with a label inside, such as "0/30" indicating character count, you can use HTML, Bootstrap and JavaScript. Here's a basic example:
This is the code...
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta name="description" content="">
<meta name="author" content="">
<meta name="generator" content="Hugo 0.84.0">
<title>sample code</title>
<!-- Bootstrap core CSS -->
<link href="css/bootstrap.min.css" rel="stylesheet">
<style>
.character-counter {
position: relative;
width: 100%; /* Adjust as needed */
}
.character-counter input[type="text"] {
width: 100%;
padding-right: 70px; /* Adjust based on your design */
}
.character-counter .count-label {
position: absolute;
top: 70%;
right: 20px;
transform: translateY(-50%);
color: gray;
font-size: 12px;
}
</style>
</head>
<body style="background-color: #EB984E;">
<main class="container" style=";margin-top: 2%;">
<div class="card border-primary mb-3" style="max-width:100%;">
<div class="card-header">www.coding30.com form here...</div>
<div class="card-body text-primary">
<div class="form-group character-counter">
<label for="exampleInputName">Enter Name</label>
<input type="text" class="form-control" id="exampleInputName" aria-describedby="emailHelp" placeholder="Enter Name...">
<div id="exampleInputName_count" class="count-label">0/30</div>
</div>
</div>
</div>
</div>
</main>
<script type="text/javascript" src="js/bootstrap.min.js"></script>
<script>
const maxCharacters_heading = 30;
const exampleInputName_input = document.getElementById('exampleInputName');
const exampleInputName_count = document.getElementById('exampleInputName_count');
exampleInputName_input.addEventListener('input', function () {
// Get the current character count
const currentCharacters = exampleInputName_input.value.length;
const remainingCharacters = maxCharacters_heading - currentCharacters;
if(remainingCharacters>-1)
{
exampleInputName_count.textContent = `${remainingCharacters}` + "/30";
}
if (currentCharacters >= maxCharacters_heading) {
exampleInputName_input.value = exampleInputName_input.value.substring(0, maxCharacters_heading);
}
});
</script>
</body>
</html>
Conclusion:
- This code creates an input field with a label inside it showing the character count out of a maximum of 30 characters. The JavaScript function is called whenever the input field changes, updating the count dynamically. The styling is basic; you can adjust it to fit your design requirements.